This is a lightning fast and extremely easy to understand guide that will teach you how to encode and decode Base32 in a Python environment in no time. We don’t just talk theory, but demonstrate the operations through a concrete example.
What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. It was created by Guido van Rossum and first released in 1991. Python has become one of the most popular programming languages in the world due to its versatility and wide range of applications.
A quick summary of Python:
- Versatile & Easy to Learn: Its simple syntax makes it an excellent introductory language, but it is also capable of handling sophisticated applications.
- General-Purpose: Used in web development, data science, scripting, machine learning, and other applications.
- Readable & Clean: Code stresses obvious structure through indentation, which improves readability and maintainability.
- Large Community & Resources: Extensive libraries and frameworks (like Django, TensorFlow) and a big online community for support.
- Rapid Prototyping: Because of its clarity and conciseness, it enables rapid idea formulation and testing.
What is Base32?
Base32 is a binary-to-text encoding scheme that represents binary data as a string of 32 different printable ASCII characters. It is often used to encode data in a human-readable format, and it’s particularly useful when the binary data needs to be shared or stored in a text-based format, such as in URLs or QR codes.
The Base32 encoding system typically uses the characters A-Z and 2-7, excluding characters that may be easily confused, like 0 and 1. This ensures that Base32-encoded strings are relatively error-resistant when manually transcribed or transmitted. The case of letters (uppercase or lowercase) may or may not be significant, depending on the specific Base32 variant.
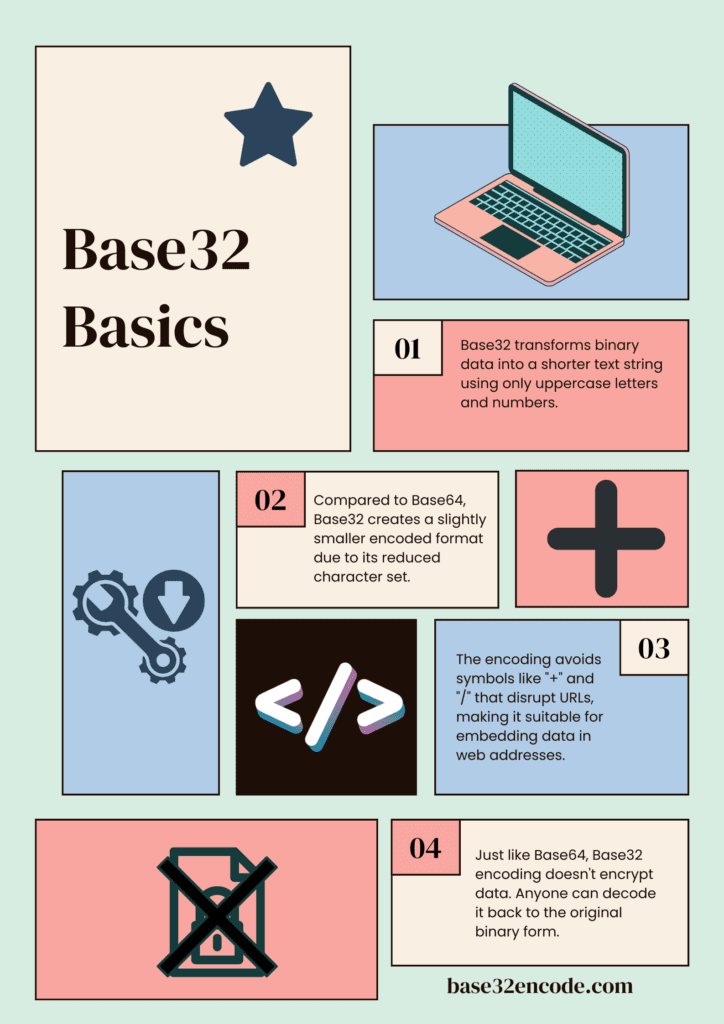
Does Python Natively Support Base32?
Python supports Base32 encoding and decoding natively. The base64
module in Python provides functions for encoding binary data to printable ASCII characters and decoding such encodings back to binary data. It provides encoding and decoding functions for the encodings specified in RFC 4648, which defines the Base16, Base32, and Base64 algorithms, and for the de-facto standard Ascii85 and Base85 encodings. You can use the base64.b32encode()
and base64.b32decode()
functions to encode and decode Base32 data in Python.
Example: Base32 Encoding & Decoding in Python
Base32 encoding and decoding is the easiest task in the world in Python, no complicated code is needed, just import a module and start encoding and decoding.
import base64 # Encoding a string to Base32 string = "Base32Encode.com" encoded = base64.b32encode(string.encode("utf-8")) print("Encoded string:", encoded.decode("utf-8")) # Decoding a Base32 string decoded = base64.b32decode(encoded) print("Decoded string:", decoded.decode("utf-8"))
This code uses the base64
module in Python to encode a string to Base32 and decode a Base32 string back to the original string. The base64.b32encode()
function encodes the string to Base32, and the base64.b32decode()
function decodes the Base32 string back to the original string. The string.encode("utf-8")
method is used to convert the string to bytes before encoding it to Base32, and the decoded.decode("utf-8")
method is used to convert the decoded bytes back to a string.