In this blog post, we will explore the fundamentals of Base32, introduce you to the Go programming language, and discuss its native support for Base32. We will also provide an example of how to encode and decode Base32 in Go. Whether you’re new to Go or looking to expand your knowledge, this guide will offer valuable insights into working with Base32 in Go.
What is Base32?
Base32 is a binary-to-text encoding scheme that represents binary data in an ASCII string format. This encoding helps to ensure that the data remains intact without modification during transport. Base32 is primarily used in situations where data needs to be stored and transferred over media that are designed to deal with text.
The Base32 encoding technique is defined as a set of 32 numbers, each represented by 5 bits of data. The exact set of 32 digits used to represent the data varies, although the most popular is stated in RFC 4648. It has an alphabet of A-Z and 2-7. The numbers 0, 1, and 8 are skipped because they are related to the letters O, I, and B.
Here’s how it works: each group of 5 bits in the data is replaced by the corresponding digit from the Base32 alphabet. Padding characters are added to the output to make its length a multiple of 8, if necessary.
One advantage of Base32 encoding is that it is reasonably user-friendly. The encoded data is case-insensitive and excludes readily misunderstood characters like ‘0’ and ‘O’. This makes it easier to use in situations where the data may need to be read or entered manually.
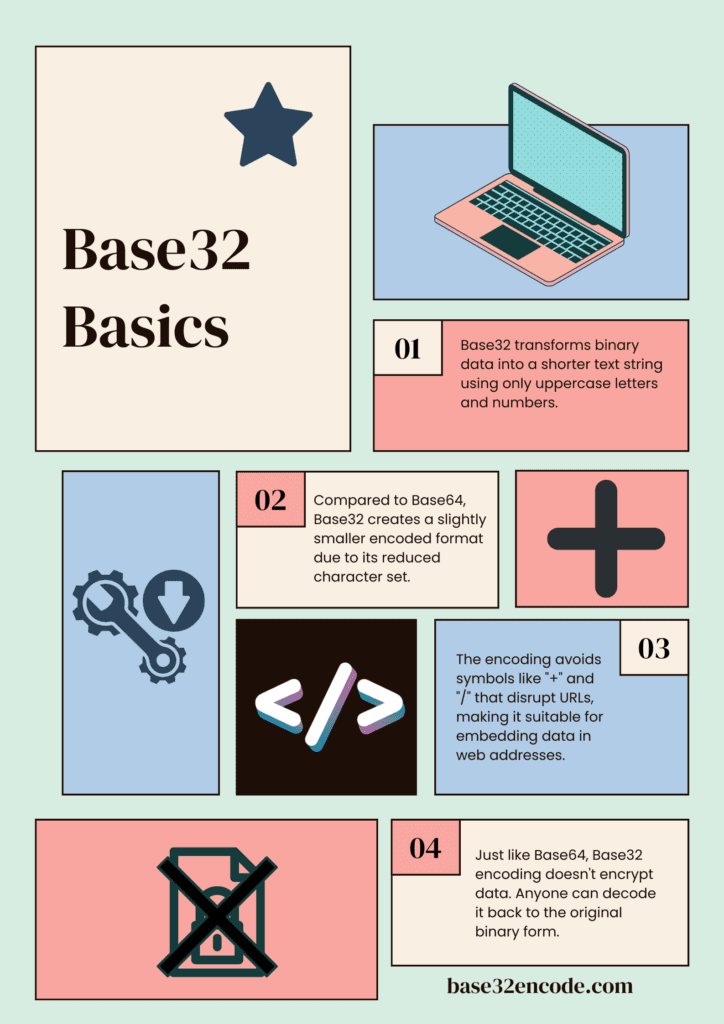
What is Go or Golang?
Go, also known as Golang, is a statically typed, compiled programming language designed at Google by Robert Griesemer, Rob Pike, and Ken Thompson. Launched in 2007, Go was designed to be simple, reliable, and efficient for software development.
Go is known for its simplicity and its ability to handle multicore, networked, and large codebases efficiently. It has a clean syntax, making it easy to learn and read. The language provides excellent support for concurrent programming, with features like goroutines and channels, which allow developers to write concurrent code directly.
Go’s standard library has a plethora of useful built-in functions for dealing with basic types. It also provides packages for web server programming, cryptography, JSON reading and writing, and more.
One of the key features of Go is its strong support for concurrent programming. Concurrency, the ability of different parts or units of a program, algorithm, or problem to be executed out-of-order or in partial order, without affecting the final outcome, is directly supported in Go, making it easier to write reliable, efficient, concurrent code.
Go also has a robust package management system. The go get command allows you to obtain, build, and install packages from remote repositories. This allows you to easily handle dependencies in your Go projects.
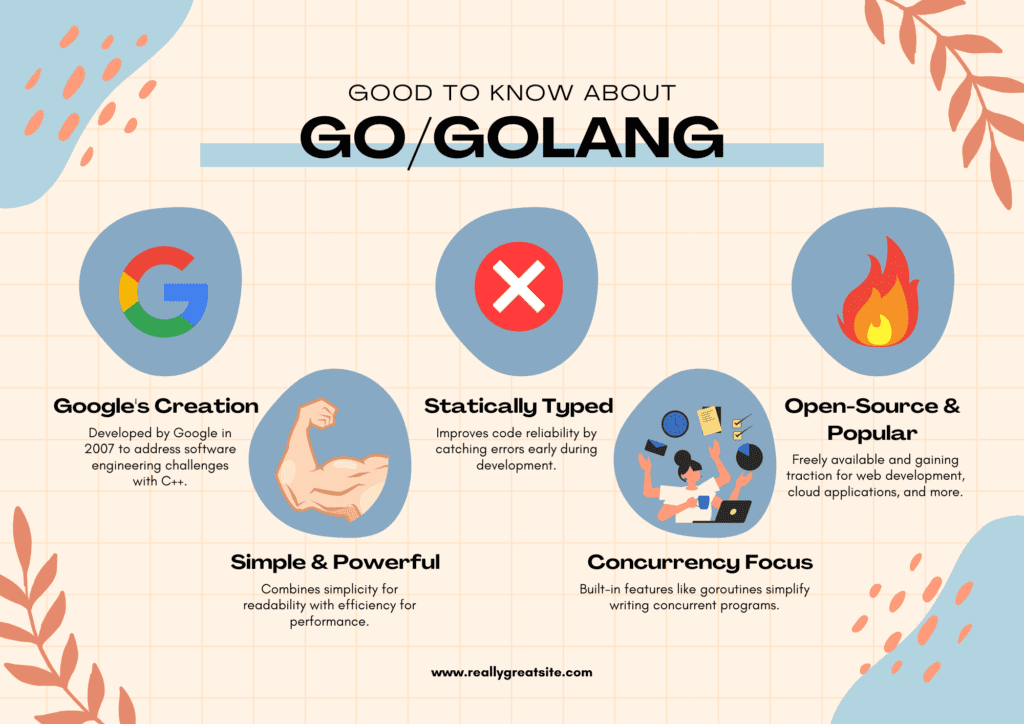
Does Go (or Golang) Natively Support Base32?
When it comes to Base32 encoding and decoding, Go has got you covered. Unlike some other languages, Go natively supports Base32. This means that you don’t need to install any additional libraries or packages to work with Base32 in Go. The encoding/base32
package in Go’s standard library provides functions for Base32 encoding and decoding. This package implements Base32 encoding as specified by RFC 4648. The package provides functions for encoding byte data to Base32 strings and decoding Base32 strings to byte data.
So the answer is yes, Golang natively supports the Base32 implementation, you need to use the encoding/base32
package, which contains the following functions:
EncodeToString()
DecodeString()
Example of Base32 Encoding and Decoding in Go
Okay, now that we know that Golang natively supports Base32, and which packages and functions it supports, it’s time to look at a concrete example of how to use it.
package main import ( "encoding/base32" "fmt" ) func main() { // The data you want to encode data := []byte("Base32Encode") // Encode the data encodedData := base32.StdEncoding.EncodeToString(data) fmt.Println("Encoded data:", encodedData) // Decode the data decodedData, _ := base32.StdEncoding.DecodeString(encodedData) fmt.Println("Decoded data:", string(decodedData)) }
In this example, we first import the encoding/base32
package. We then use the EncodeToString
function to encode byte data to a Base32 string, and the DecodeString
function to decode a Base32 string to byte data.
So, if you’re working with Go and need to encode or decode data in Base32, you can use the encoding/base32
package that’s included in the standard library. It’s efficient, easy to use, and doesn’t require any external dependencies.