Dive into our detailed guide on Base32 in PHP. Learn what Base32 is, explore PHP’s native support, discover top Base32 libraries, and even create your own implementation. Perfect for beginners and experts alike.
What is Base32?
Base32 is a way of representing binary data using a set of 32 characters. Imagine translating a long string of 1s and 0s into a more user-friendly alphabet. This makes data easier to read, transmit, and manage.
There are several advantages to using Base32 encoding:
- Enhanced Readability: Unlike raw binary, Base32 allows us to view the data. This makes it easier to find mistakes, communicate data, and even debug code.
- Space Efficiency: Base32 has a somewhat bigger alphabet than base-10 (numbers 0-9), but it is still more compact than binary data. This can be useful when working with limited storage space.
- URL-friendliness: The Base32 character set excludes symbols that could cause issues in web addresses or other text-based systems. This makes it appropriate for embedding binary data into URLs or text files.
Base32 encoding finds use in a variety of scenarios:
- Shortening Identifiers: Long IDs used in file systems or databases can be significantly shortened using Base32 encoding, making them easier to manage and share.
- Data Transmission: When transferring binary data over channels that only allow text forms, Base32 can be a handy tool.
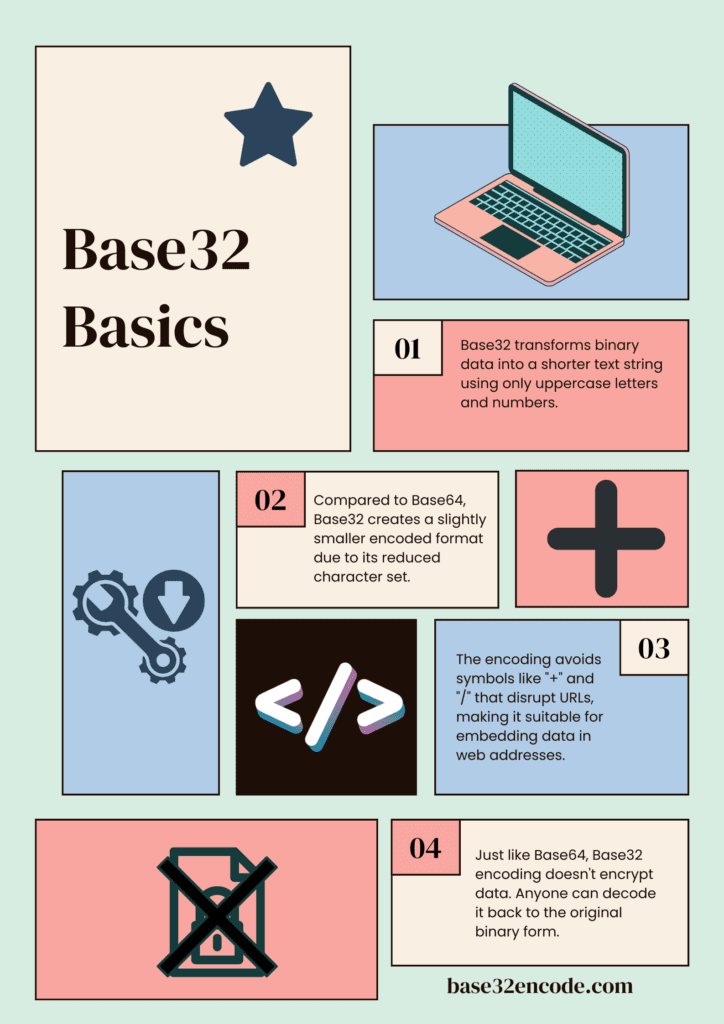
What is PHP? Does it Natively Support Base32?
Born in 1994 from the mind of Rasmus Lerdorf, PHP initially aimed to simplify managing personal homepages. Over time, it evolved into a robust language capable of driving some of the most popular websites in the world.
PHP, which stands for Hypertext Preprocessor, is a versatile scripting language used for web development and server-side programming. It offers various functions and libraries to handle data encoding and decoding tasks.
PHP provides a plethora of functions and libraries for developers to work with, but does it natively support Base32 encoding and decoding? PHP does not inherently provide native support for Base32 encoding and decoding.
Here’s what makes PHP a powerful web development tool:
- Server-Side Scripting: Unlike HTML, which specifies the structure of a web page, PHP is a server-side scripting language. This means that PHP code runs on the web server before the content is delivered to the user’s browser. This enables dynamic content development, database interaction, and individualized user experiences.
- Ease of Use: PHP’s syntax is generally straightforward, making it easier to learn than some other programming languages. This helps developers to focus on creating online applications rather than getting mired down in intricate coding structures.
- Versatility: PHP is a general-purpose programming language that can be used for more than just web pages. It can handle activities such as form processing, email sending, and database management, making it an excellent tool for developing complex online applications.
- Large Community and Resources: Because of its popularity, PHP has a large and active development community. This translates into an abundance of online resources, tutorials, and forums, making it easier for newcomers to learn and experienced developers to solve problems.
Base32 Libraries for PHP
Leverage the power of external libraries for Base32 encoding and decoding, and save time and effort in your development process.
Thanks to the enthusiastic PHP community, you can choose from several excellent libraries to streamline your work. By importing one of these external libraries into your program, you can avoid the need to write your own Base32 code, saving time and ensuring that you benefit from well-tested and optimized solutions.
These libraries offer a range of functionalities and are easy to integrate, making them an invaluable resource for developers working with Base32 encoding and decoding in PHP.
As we said, there are several libraries available for encoding and decoding Base32 in PHP. Here are a few options:
- ademarre/binary-to-text-php: This repository handles non-standard variants of common encoding schemes like Base64 and Base32, implementing a unified algorithm.
- NTICompass/PHP-Base32: This Base32 library supports RFC 4648 and the Crockford’s implementation.
- christian-riesen/base32: This library can be added to the composer.json file.
- williameggers/php-totp: This library includes a Base32 implementation as part of a TOTP/HOTP library.
Own Base32 Implementation
Take full control of your Base32 encoding and decoding process by creating your own custom code. In scenarios where using external libraries is not feasible or when you need a tailored solution, developing your own encoder can be an empowering and valuable option.
In this section, we will first introduce you to the fundamentals of Base32 encoding and decoding. Then, we will guide you through the process of implementing your own Base32 encoder and decoder, equipping you with the knowledge and skills to create a custom solution that fits your unique requirements. By the end of this section, you will have a clear understanding of how Base32 works and be able to implement your own encoder and decoder in your preferred programming language.
Base32 Characters
Understanding the Base32 character set is critical for developing your own Base32 encoder and decoder. The Base32 character set has 32 characters, including uppercase letters A-Z and numbers 2-7. This carefully chosen set eliminates the requirement for special characters, making Base32 more acceptable for usage in URLs and filenames than Base64, which requires URL encoding.
Understanding the Base32 character set will prepare you to design your own encoder and decoder capable of efficiently and accurately handling Base32 encoding and decoding operations.
The Base32 character set consists of 32 characters:
- Uppercase letters A to Z (26 characters)
- Digits 2 to 7 (6 characters)
How Base32 works?
Here are the steps to follow when encoding Base32:
- Divide the binary data into 40-bit (8-character) chunks. If the data’s length is not a multiple of 40 bits, you will need to handle padding.
- Convert each 40-bit chunk into its equivalent Base32 representation by mapping each 5-bit segment to its corresponding character from the character set.
- Append padding characters as necessary to ensure that the output is a multiple of 8 characters.
- The result is a Base32-encoded string, ready for transmission, storage, or further use.
Decoding involves the same steps, but in the opposite direction, i.e. backwards.
Language Independent Base32 Implementation (Pseudo Code)
Now we will demonstrate the Base32 encoding implementation using pseudo code.
function base32_encode(data): // Define the Base32 alphabet alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ234567' // Convert the input data to binary binary_data = ''.join(format(ord(char), '08b') for char in data) // Pad the binary data with zeros if necessary while len(binary_data) % 5 != 0: binary_data += '0' // Split the binary data into 5-bit chunks chunks = [binary_data[i:i+5] for i in range(0, len(binary_data), 5)] // Convert each 5-bit chunk to its corresponding Base32 character encoded_data = ''.join([alphabet[int(chunk, 2)] for chunk in chunks]) return encoded_data
This implementation accepts a string of data as input and returns the Base32-encoded string. It works by converting the incoming data to binary and then dividing it into 5-bit pieces. Each 5-bit chunk is then transformed to a Base32 character using the Base32 alphabet. Finally, a Base32-encoded string is returned.
This implementation assumes that the incoming data is a string of characters. If the input data is in a different format, such as a byte array, the implementation must be adjusted accordingly.
Finally, let’s look at the decoding process:
function base32_decode(encoded_data): // Define the Base32 alphabet alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ234567' // Convert the encoded data to binary binary_data = ''.join([format(alphabet.index(char), '05b') for char in encoded_data]) // Pad the binary data with zeros if necessary while len(binary_data) % 8 != 0: binary_data += '0' // Split the binary data into 8-bit chunks chunks = [binary_data[i:i+8] for i in range(0, len(binary_data), 8)] // Convert each 8-bit chunk to its corresponding ASCII character decoded_data = ''.join([chr(int(chunk, 2)) for chunk in chunks]) return decoded_data