In this guide, we will explore the basics of C# and Base32 encoding, including how to encode and decode Base32 data in C#. We will also discuss the various Base32 libraries available for C# and how to implement your own Base32 encoder and decoder.
What is C#?
C# (pronounced “C sharp”) is a modern, high-level, object-oriented programming language developed by Microsoft. It was introduced in the early 2000s as a part of the Microsoft .NET platform. C# is designed for building a wide range of software applications, including desktop applications, web applications, games, mobile apps (via Xamarin), and more.
C# is an object-oriented programming language, which means it is based on the concept of objects and classes, promoting code organization and reusability.
C# has become a popular choice for building Windows applications, web applications, and cross-platform mobile applications. It’s known for its ease of use, powerful development tools (such as Visual Studio), and extensive documentation.
Here’s a short breakdown about C#:
- General-purpose: It can be used for various tasks, from building websites and games to creating complex enterprise applications.
- High-level: C# code is closer to human language than machine code, making it easier to read, write, and maintain.
- .NET platform: C# is tightly integrated with the .NET framework, providing a vast library of tools and functionalities for developers.
What is Base32?
Base32 is a binary-to-text encoding scheme that represents binary data as a string of 32 different printable ASCII characters. It is often used to encode data in a human-readable format, and it’s particularly useful when the binary data needs to be shared or stored in a text-based format, such as in URLs or QR codes.
The Base32 encoding scheme typically uses the characters A-Z and 2-7, excluding easily confused characters such as 0 and 1. This ensures that Base32 encoded strings are relatively error-free when manually transcribed or transmitted.
There are several different adaptations of Base32, each differing in a few minor details, such as the character set. Some use lower case instead of upper case, some include some characters that others do not, and some omit some.
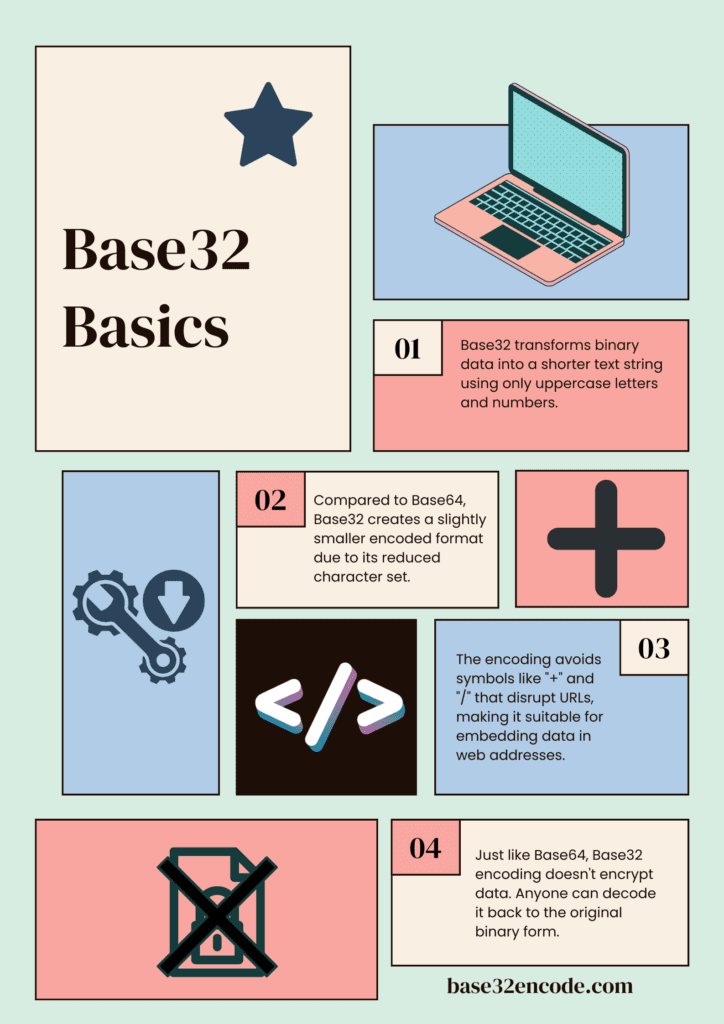
Base32 Encoding & Decoding in C#
C# does not natively support Base32 encoding and decoding in the .NET Framework. However, you can work with Base32 encoding in C# by using third-party libraries or implementing your own Base32 encoding and decoding functions.
If you’re wondering what libraries are, here’s the answer: a library is a collection of pre-written code, functions, classes, or modules that can be used by software developers to perform common tasks or add specific functionality to their applications. Libraries provide a way to reuse code, save time, and avoid reinventing the wheel for commonly needed features or operations. They can be used in various programming languages, including C#, Java, Python, and many others.
Base32 Libraries for C#
There are various third-party libraries available for handling Base32 encoding and decoding in C#, such as:
- base32: This library implements a Base32 encoder and decoder based upon the RFC 4648 standard.
- crockford-base32-core: This is a library that provides Crockford’s Base32 encoding and decoding functionality.
Own implementation
If the use of external libraries is not possible, or if you need full control over the coding process, or if you just want to practice, creating your own Base32 encoder is a real option.
This section will introduce you to the basics of Base32, guide you through the steps of creating your own Base32 encoder and help you to make the implementation easier and more transparent.
Base32 Characters
To implement Base32, we first need to get to know the character set, without which it is impossible to implement it. The Base32 character set consists of 32 characters, which contain the following according to the standard Base32:
- Uppercase letters: A-Z (26 characters)
- Digits: 2-7 (6 characters)
While Base64’s character set contains special characters that can be problematic in certain cases and contexts, such as URLs and filenames. Base32, on the other hand, does not contain any special characters, so it is perfectly applicable in the above cases.
How Base32 works?
Here are the steps to follow when encoding Base32:
- Divide the binary data into 40-bit (8-character) chunks. If the data’s length is not a multiple of 40 bits, you will need to handle padding.
- Convert each 40-bit chunk into its equivalent Base32 representation by mapping each 5-bit segment to its corresponding character from the character set.
- Append padding characters as necessary to ensure that the output is a multiple of 8 characters.
- The result is a Base32-encoded string, ready for transmission, storage, or further use.
During decoding, we have to follow the same sequence of operations, but in the opposite order.
Language Independent Base32 Implementation (Pseudo Code)
Now we will demonstrate the Base32 encoding implementation using pseudo code.
function base32_encode(data): // Define the Base32 alphabet alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ234567' // Convert the input data to binary binary_data = ''.join(format(ord(char), '08b') for char in data) // Pad the binary data with zeros if necessary while len(binary_data) % 5 != 0: binary_data += '0' // Split the binary data into 5-bit chunks chunks = [binary_data[i:i+5] for i in range(0, len(binary_data), 5)] // Convert each 5-bit chunk to its corresponding Base32 character encoded_data = ''.join([alphabet[int(chunk, 2)] for chunk in chunks]) return encoded_data
This function converts input data to binary and then splits it into 5-bit chunks. Each chunk is then converted to its corresponding Base32 character using the Base32 alphabet. The resulting Base32-encoded string is returned.
It is important to note that this function is designed to work with input data that is a string of characters. If the input data is in a different format, such as a byte array, the implementation must be modified accordingly.
Finally, let’s look at the decoding process:
function base32_decode(encoded_data): // Define the Base32 alphabet alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ234567' // Convert the encoded data to binary binary_data = ''.join([format(alphabet.index(char), '05b') for char in encoded_data]) // Pad the binary data with zeros if necessary while len(binary_data) % 8 != 0: binary_data += '0' // Split the binary data into 8-bit chunks chunks = [binary_data[i:i+8] for i in range(0, len(binary_data), 8)] // Convert each 8-bit chunk to its corresponding ASCII character decoded_data = ''.join([chr(int(chunk, 2)) for chunk in chunks]) return decoded_data