In this tutorial, we will explore how to do Base32 coding in JavaScript (native and Node), with and without an external library. Specific external libraries will be offered and shown how to use them, as well as how to implement Base32 without external libraries in a JavaScript environment.
What is Base32 Encoding?
Base32 is a binary-to-text encoding scheme that allows you to represent binary data using a set of 32 distinct characters. It is particularly valuable in scenarios where data needs to be shared or stored in a text format, such as in URLs, file and folder names, and cryptographic applications. Base32 operates by dividing binary data into 5-bit groups and mapping each group to a corresponding character from its predefined character set.
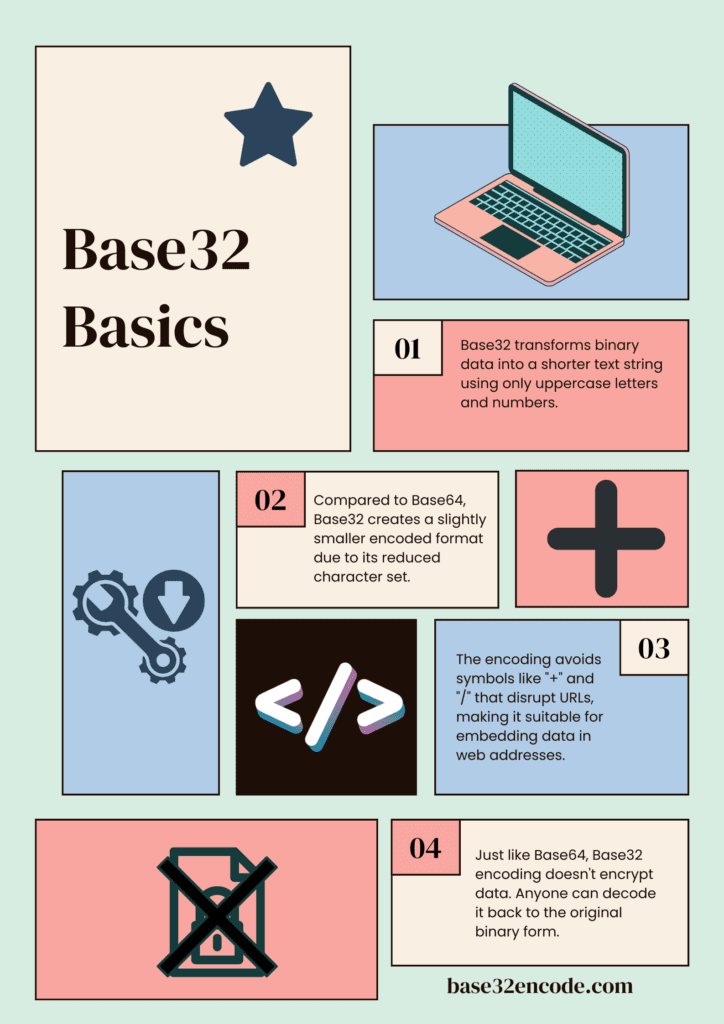
What is JavaScript and Node.js?
JavaScript is a versatile, high-level programming language commonly used for web development. Unlike Java, they are distinct languages, although the name “JavaScript” can be misleading. JavaScript is primarily employed for client-side scripting in web browsers, enabling web pages to become interactive and dynamic. It provides the means to manipulate the Document Object Model (DOM), respond to user input, and communicate with web servers using AJAX (Asynchronous JavaScript and XML) requests. JavaScript is an essential component of modern web development, making web applications more engaging and responsive. It has a broad ecosystem of libraries and frameworks, such as React, Angular, and Vue.js, that simplify the development of complex web applications.
Node.js is an open-source, server-side runtime environment that is built on the V8 JavaScript engine. It allows developers to execute JavaScript code on the server, enabling them to build scalable and high-performance network applications. Node.js is particularly well-suited for building web applications, APIs, and other networked software.
Does JavaScript or Node.js Natively Support Base32? Base32 Encoding in JavaScript
JavaScript and Node.js, as they stand, do not have built-in support for Base32 encoding. However, there are plenty of third-party libraries available to add Base32 encoding to your JavaScript projects. Alternatively, you can implement your own. Either way, we can help you implement both.
Base32 Libraries for JavaScript/Node.js
In this section, we will introduce you to some of the most popular and reliable JavaScript/Node.js libraries available for Base32 encoding, which can help you save time and effort in your development projects.
We will also look at a number of third-party libraries that offer Base32 encoding functionality, highlighting their key features and benefits.
base32-js
This library provides a simple API for encoding and decoding Base32 strings in JavaScript. It is based (loosely) on Crockford’s Base32 and is intended to be human-friendly.
Installing it is also very simple, just install it in Shell with the following command:
npm install base32
You can then use it in your program as follows:
var base32 = require('base32') var encoded = base32.encode('some data to encode') var decoded = base32.decode(encoded)
hi-base32
This is a simple Base32 encode/decode function for JavaScript that supports UTF-8 encoding. It provides a simple API and is easy to use.
bower install hi-base32 // OR npm install hi-base32
You can then use it for Base32 encoding/decoding:
var base32 = require('hi-base32'); base32.encode('String to encode'); base32.decode('Base32 string to decode');
Own implementation
If using external libraries is not possible or if you require complete control over the encoding process, creating your own Base32 encoder is a viable option.
This section will introduce the basics of Base32 and guide you through the process of implementing your own encoder and decoder.
Base32 Characters
In order to understand exactly how Base32 works, we first need to familiarise ourselves with its character set, which is essential for its implementation. The Base32 character set consists of 32 characters, which typically include:
- Uppercase letters A to Z (26 characters)
- Digits 2 to 7 (6 characters)
Unlike Base64, Base32 does not include special characters that might cause issues in certain contexts, making it suitable for use in URLs and filenames without the need for URL encoding.
How Base32 works?
Follow these steps to encode in Base32:
- Divide the binary data into 40-bit (8-character) chunks. If the data’s length is not a multiple of 40 bits, you will need to handle padding.
- Convert each 40-bit chunk into its equivalent Base32 representation by mapping each 5-bit segment to its corresponding character from the character set.
- Append padding characters as necessary to ensure that the output is a multiple of 8 characters.
- The result is a Base32-encoded string, ready for transmission, storage, or further use.
During decoding, the same sequence of operations must be followed, but in reverse order.
Language Independent Base32 Implementation (Pseudo Code)
We will now demonstrate the implementation of Base32 encoding using pseudocode.
function base32_encode(data): // Define the Base32 alphabet alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ234567' // Convert the input data to binary binary_data = ''.join(format(ord(char), '08b') for char in data) // Pad the binary data with zeros if necessary while len(binary_data) % 5 != 0: binary_data += '0' // Split the binary data into 5-bit chunks chunks = [binary_data[i:i+5] for i in range(0, len(binary_data), 5)] // Convert each 5-bit chunk to its corresponding Base32 character encoded_data = ''.join([alphabet[int(chunk, 2)] for chunk in chunks]) return encoded_data
This function converts input data to binary and then splits it into 5-bit chunks. Each chunk is then converted to its corresponding Base32 character using the Base32 alphabet. The function ultimately returns a string that has been encoded in Base32.
Note that this implementation assumes that the input data is a string of characters. If the input data is in a different format, such as a byte array, the implementation would need to be modified accordingly.
Finally, let’s look at the decoding process:
function base32_decode(encoded_data): // Define the Base32 alphabet alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ234567' // Convert the encoded data to binary binary_data = ''.join([format(alphabet.index(char), '05b') for char in encoded_data]) // Pad the binary data with zeros if necessary while len(binary_data) % 8 != 0: binary_data += '0' // Split the binary data into 8-bit chunks chunks = [binary_data[i:i+8] for i in range(0, len(binary_data), 8)] // Convert each 8-bit chunk to its corresponding ASCII character decoded_data = ''.join([chr(int(chunk, 2)) for chunk in chunks]) return decoded_data